Reading and Writing Binary data through fstream
- Lakhan Dhakad
- May 14, 2020
- 2 min read
C++ file input and output are typically achieved by using an object of one of the following classes:
ifstream for reading input only.
ofstream for writing output only.
fstream for reading and writing from/to one file.
All three classes are defined in <fstream.h>. Throughout this page, the term "file stream" will be used when referring to features that apply equally to all three classes.
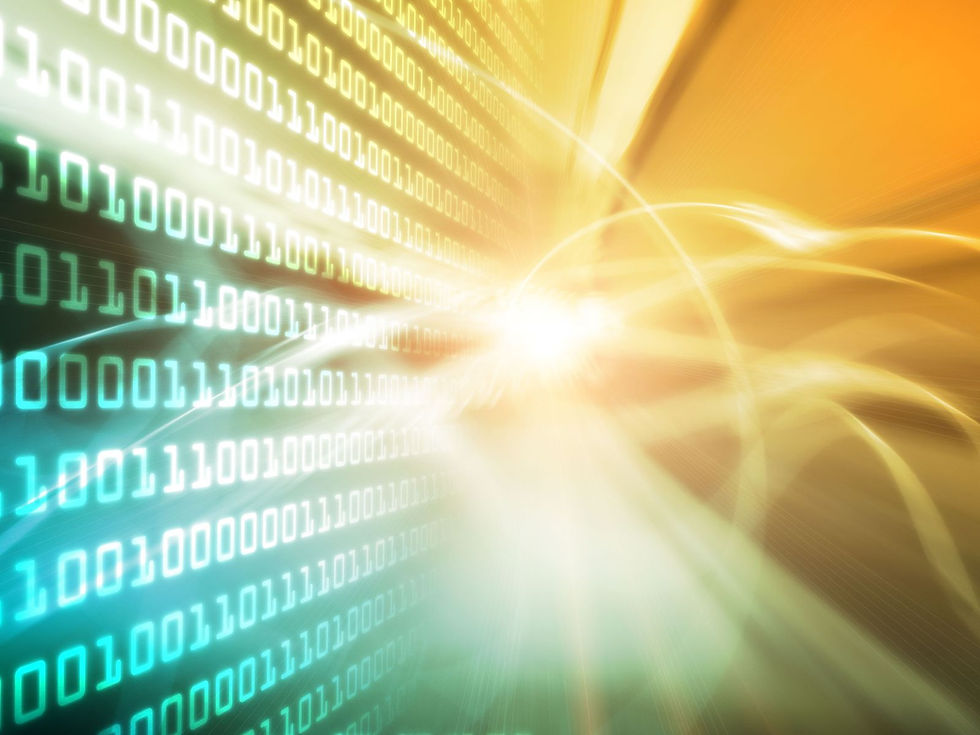
include<iostream.h>
#include<conio.h>
#include<fstream.h>
#include<stdio.h>
char choice,name[10];
int r;
class student
{
int rno;
char name[30];
float marks;
public:
void take()
{
cout<<"\nEnter roll no. :";
cin>>rno;
cout<<"\nEnter name :";
gets(name);
cout<<"\nEnter marks :";
cin>>marks;
}
void put()
{
cout<<"\nRoll no.:"<<rno;
cout<<"\nName is:"<<name;
cout<<"\nMarks is:"<<marks;
}
int rnr(){
return rno;
}
}obj;
void reading()
{
cout<<"Enter name of file ";
gets(name);
ofstream fout;
fout.open(name,ios::out,ios::binary);
do
{
obj.take();
fout.write((char*)&obj,sizeof(obj));
cout<<"Want to enter more?(y/n):";
cin>>choice;
}while(choice=='y');
fout.close();
}
void opening()
{
cout<<"Enter name of file ";
gets(name);
ifstream fin;
fin.open(name,ios::in,ios::binary);
while(fin.read((char*)&obj,sizeof(obj)))
{
obj.put();
}
fin.close();
}
void search()
{
cout<<"Enter name of file ";
cin>>name;
ifstream fin;
fin.open(name,ios::in,ios::binary);
cout<<"\nEnter roll no. to be searched :";
cin>>r;
fin.seekg(0);
while(fin.read((char*)&obj,sizeof(obj)))
{
if(obj.rnr()==r)
{
obj.put();
break;
}
}
fin.close();
}
void modify()
{
cout<<"\nEnter name of file :";
gets(name);
ifstream fin;
fin.open(name,ios::in||ios::binary);
ofstream fout;
fout.open(name,ios::out||ios::binary);
cout<<"\nEnter roll no. to be modified :";
cin>>r;
while(fin.read((char*)&obj,sizeof(obj)))
{
int pos=fin.tellg();
if(obj.rnr()==r)
{
obj.take();
fout.seekp(pos-sizeof(obj));
fout.write((char*)&obj,sizeof(obj));
break;
}
}
fout.close();
fin.close();
}
void dell()
{
cout<<"\nEnter name of file :";
gets(name);
ifstream fin;
fin.open(name,ios::in||ios::binary);
ofstream fout;
fout.open("temp.txt",ios::out||ios::binary);
cout<<"\nEnter roll no. to be deleted:";
cin>>r;
fin.seekg(0);
while(fin.read((char*)&obj,sizeof(obj)))
{
if(obj.rnr()!=r)
fout.write((char*)&obj,sizeof(obj));
}
fout.close();
fin.close();
remove(name);
rename("temp.txt",name);
}
void main()
{
clrscr();
char ch;
int o;
do{
clrscr();
cout<<"\nTo read:1\nTo open:2\nTo search:3\nTo modify:4\nTo Delete:5 ---";
cin>>o;
switch(o)
{
case 1:reading();
break;
case 2:opening();
break;
case 3:search();
break;
case 4:modify();
break;
case 5:dell();
break;
}
cout<<"\n\nWnat to continue?(y/n):";
cin>>ch;
}while(ch=='y');
getch();
}
Follow Programmers Door for more.
Comentarios