Structure of a C Program
- Shuchi Vyas
- Aug 4, 2020
- 4 min read
In this blog you will learn about the structure of a program in C. The previous blog was about an introduction to the C language, which you can find here.
As we have learned, C is a middle-level, procedure oriented programming language. This means that unlike object oriented languages like Java, in which programs are organised around a set of classes and objects, the programs in C are organised around a set of functions. The structure of a program in C is, thus, much simpler than that in Java.
All C programs follow a structure, which is as follows:
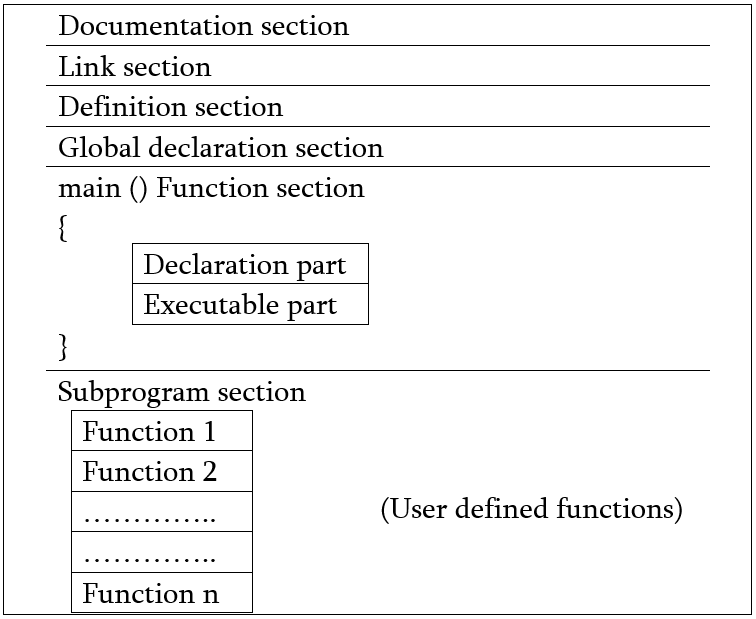
Any program in the C language is divided into six sections - the documentation section, the link section, the definition section, the global declaration section, the main() function section and the subprogram section. Let's discuss all of them one by one:
Documentation Section - The first section of a C program is the documentation section. It is a set comment lines where information like the name of the program, name of the author, etc. are mentioned, which can be used by other programmers to identify the code.
Link Section - The link section of a C program consists of instructions to the compiler to link the header files or functions from the system.
Definition Section - The third section is the definition section which contains symbolic constants. Generally, the preprocessor directive '#define' is used to create constants.
Global Declaration Section - Global declaration section is where global variables or user defined functions are declared. Global variables are the variables that can be used in more than one function; whereas local variables can only be used in the function they are declared in.
Main Function Section - This section contains the main function of the program. Every program must have one main() function.
Subprogram Section - The subprogram section is where the user-defined functions are defined, in case the program is a multi-function program. These user-defined functions can be called in the main() function or in each other.
Let’s try to understand the structure with help of an example. In the following program, we are trying to find the area of a circle.
/* areaofcircle.c
author: shuchi
date: 04-08-2020
*/
#include<stdio.h>
#define PI 3.14
float area(int r);
int main()
{ int r;
printf("Enter the value of radius \n");
scanf("%d",r);
float a;
a=area(r);
printf("The area of the circle is %f",a);
return 0;
}
float area(int r)
{ float a;
a = PI*r*r;
return a;
}
Now let’s study the sections of the above program.
/* areaofcircle.c
author: shuchi
date: 04-08-2020
*/
This is the documentation section of the program. In C, we can create comments using ‘//’ for single line comments, or using ‘/* */’. Comments are ignored by the compiler.
#include<stdio.h>
This is the link section of the program, which contains commands to link header files into the program. Some common header files which are used are stdio.h, conio.h, math.h, etc.
#define PI 3.14
This is the definition section of the program. Here we have defined a constant ‘pi’ and assigned the value of 3.14 to it. Remember, the '#define' is not a statement and must not end with a semicolon (;).
float area(int r);
This is the global declaration section of the program. Here we have declared the function ‘area’. It is a user-defined function which takes the value of the radius, of the datatype int, as argument and calculates the area of the circle.
int main()
{ int r;
printf("Enter the value of radius \n");
scanf("%d",r);
float a;
a=area(r);
printf("The area of the circle is %f",a);
return 0;
}
This is the main() function of the program. In the main function we have asked the user to enter the value of the radius. Then the user-defined function ‘area’ is called and the value of the radius is passed as argument to it. The function returns the calculated area of the circle. We can print out the result using the ‘printf’ function.
float area(int r)
{ float a;
a = PI*r*r;
return a;
}
This is the subprogram section of the program. Here we are defining the function ‘area’ that we previously declared in the global declaration section. This function simply calculates the area of the circle using the formula ‘pi * r * r’ and returns it.
Keep in mind that this function has been mentioned three times in the program. The first time was in the global declaration section, where the function is merely declared, i.e., the compiler is made aware of the existence of the function, its name, return type and parameters. The second time was inside the main() function, which calls the ‘area’ function. You can study calling a function in later blogs. The third time was in the subprogram section where the function was defined, i.e., the body of the function was provided. A function can be declared and defined together, but it should be at least declared before it is called.
Happy coding!
Follow us on Instagram @programmersdoor
Join us on Telegram @programmersdoor
Please leave comments if you find any bug in the above code, or find other ways to solve the same problem.
Follow Programmers Door for more.
Comments