J2SE (Core Java)
- Lakhan Dhakad
- Jun 8, 2020
- 2 min read
Also known as Core Java, this is the most basic and standard version of Java.It’s the purest form of Java, a basic foundation for all other editions.
It consists of a wide variety of general purpose API’s (like java.lang, java.util) as well as many special purpose APIs
J2SE is mainly used to create applications for Desktop environment.
It consist all the basics of Java the language, variables, primitive data types, Arrays, Streams, Strings Java Database Connectivity(JDBC) and much more. This is the standard, from which all other editions came out, according to the needs of the time.
Topics that we will cover later in this series.
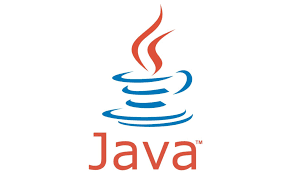
Basics of Java
Java - What, Where, and Why?
Features of Java
Introduction to JDK, JRE
Compilation Process
Java Program Structure
Java Naming Conventions
Import / Package Statements
Main method of Java
Control Flows
Data Types and Type Conversions
Comments In Java
Operators In Java
OOPS Concepts
Advantage of OOPs
Object and Class
Abstraction Encapsulation
Types of Variables (instance/class)
Static methods / non-static methods
Call by value – call by reference
Method Overloading
Var-args methods
Constructor
this keyword
Objects & References
Garbage Collection and finalize method
Inheritance
super keyword / final keyword
Abstract class and Interface
Package and Access Modifiers
Object Cloning
String Handling
String (Constructors, Methods)
Concept of Immutable and Mutable
Objects
String Pool
StringBuffer (Constructors, Methods)
StringTokenizer(Constructors, Methods)
Exception Handling
Exception Handling: What and Why?
try and catch block Multiple catch
block
Nested try
finally block
throw keyword
Exception Propagation
throws keyword
Exception Handling with Method
Overriding
Custom Exception
Input and output
File class (uses, constructors and methods)
Concept of Streams (Byte Based and Character Based)
File Output Stream & File Input Stream
Sequence Input Stream
Buffered Output Stream & Buffered Input Stream
File Writer & File Reader
Input from keyboard by Scanner
Compressing and Uncompressing File
Data Input Stream and Data Output Stream
Serialization & De-serialization
transient keyword
Collection
Collection Framework Introduction
List, Set, Queue interfaces and their implementations
Map and its implementations
Comparable and Comparator
java.util.Arrays class and its methods
Single Dimension Arrays (primitive and non-primitive types)
Multi Dimension Arrays (2d,3d)
Multithreading
Multithreading: What and Why?
Life Cycle of a Thread
Creating Thread
Thread Examples
Thread class methods
Synchronization
Synchronized Blocks and methods
Inter-thread communication
Runnable interface
GUI Designing and Event
Handling
Containers & Components
Layout Managers
Methods of Component classes
Intermediate Container
Font, Color classes
Dialogs, File Dialogs
Event Handling
Event Handling using Listeners
Event Handling using Adapter classes
Event Handling using inner and Anonymous classes
Java Applets
Happy Coding! Follow us on Instagram @programmersdoor Join us on Telegram @programmersdoor Please write comments if you find anything incorrect, or you want to share more information about the topic discussed. Follow us on Instagram and telegram for more updates #programming #blog
Comments